Top 50 PHP Interview Questions and Answers
- IOTA ACADEMY
- Apr 9
- 7 min read
One of the most popular server-side scripting languages for web development is PHP (Hypertext Preprocessor). It is renowned for being easy to use, adaptable, and database-integrable. Regardless of your level of experience, you must have a firm grasp of PHP's foundational ideas, sophisticated concepts, and practical applications in order to prepare for an interview.
This blog offers 50 frequently asked PHP interview questions along with thorough responses that cover fundamental, intermediate, and advanced subjects.
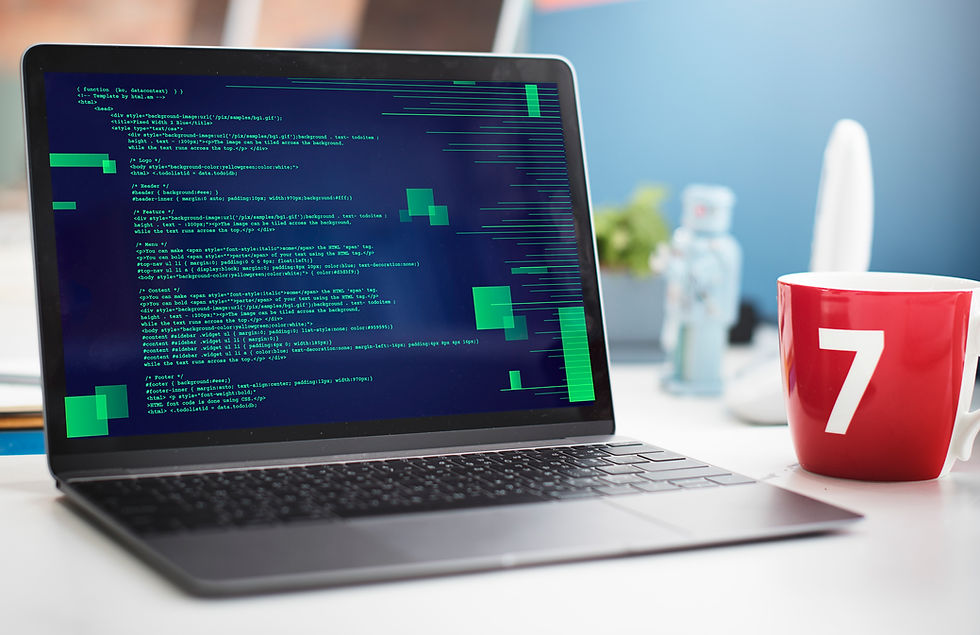
Basic PHP Interview Questions
1. What is PHP?
PHP is a server-side scripting language primarily used for web development. It creates dynamic web pages by running on the server and being integrated within HTML. Rasmus Lerdorf invented it in 1994. PHP is open-source, compatible with a number of databases, and easily combines with JavaScript, HTML, and CSS.
The general-purpose programming language PHP is used to create online applications and websites. It is a server-side scripting language that is integrated with HTML to create online applications, dynamic websites, and static websites.
2. What are the key features of PHP?
PHP is known for features such as:
Free to use and open-source.
Connectivity between platforms (Windows, macOS, Linux).
Incorporated into HTML.
Supports a variety of databases, including PostgreSQL and MySQL.
Features integrated cookie and session management.
3. What is the difference between PHP and JavaScript?
PHP is a server-side scripting language, meaning it executes on the server before sending the result to the browser. JavaScript, on the other hand, is a client-side language that runs directly in the user's browser to provide dynamic interactions without reloading the page.
4. How do you declare a variable in PHP?
In PHP, variables are declared using the $ symbol followed by the variable name. Unlike some languages, PHP does not require explicit data type declarations, as it is dynamically typed language.
5. What are PHP data types?
A data type defines the kind of data a variable can hold in a programming language.
PHP supports several data types, including:
String: "Hello, World!"
Integer: 42
Float (Double): 3.14
Boolean: true or false
Array: ['apple', 'banana', 'cherry']
Object: Instance of a class
NULL: Represents an empty variable
6. What is the difference between echo and print in PHP?
Both echo and print are used to display output in PHP. The main differences are:
Echo does not return a value and can produce many strings simultaneously.
Print is a little slower because it can only output a single string and returns a value of 1.
7. What are PHP constants?
Constants are variables that, once defined, cannot have their values altered. The const keyword or the define() function are used to declare them. The $ sign is not used by constants.
8. What are superglobals in PHP?
Superglobals are built-in PHP variables that are available in all scopes of a script. These include:
$_GET – Retrieves data sent via URL parameters.
$_POST – Retrieves data sent via an HTML form.
$_SESSION – Stores session data for a user.
$_COOKIE – Retrieves stored cookies.
9. What is the difference between == and === in PHP?
== checks if values are equal, performing type conversion if necessary.
=== checks both value and data type (strict comparison).
10. What is the purpose of the isset() and empty() functions?
isset($var) checks if a variable is set and not NULL.
empty($var) checks if a variable is unset, NULL, or evaluates to false.
11. What is the difference between PHP4 and PHP5?
PHP5 introduced Object-Oriented Programming (OOP) features such as classes, inheritance, polymorphism, interfaces, and constructors. It also improved error handling, XML support, and MySQLi (improved MySQL extension).
12. What is meant by a scripting language?
Programming languages that can automate processes without compilation by executing scripts at runtime are known as scripting languages. Scripting languages include PHP, Python, and JavaScript.
13. What is the default file extension of PHP files?
PHP files have the extension .php (e.g., index.php).
14. What is the difference between static and dynamic websites?
A dynamic website uses databases like MySQL and programming languages like PHP to create content dynamically, whereas a static website shows fixed content.
15. How can you display text in PHP?
Functions like echo and print can be used to display text.
16. How does PHP handle whitespace?
New lines, tabs, and additional spaces are ignored by PHP unless they are contained within strings.
17. What are magic constants in PHP?
Magic constants are built-in PHP constants that change depending on where they are used. Examples:
FILE – Returns the current file's full path.
LINE – Returns the current line number in the script.
18. What is the purpose of the header() function?
The header() function sends raw HTTP headers to the browser. It is used for redirecting pages and controlling caching.
19. What is the difference between die() and exit() in PHP?
Both die() and exit() terminate the script execution, but die() can also print a message before stopping execution.
20. What are PHP error levels?
PHP has different error levels such as:
E_WARNING: Non-fatal runtime errors.
E_NOTICE: Non-critical warnings.
E_ERROR: Fatal runtime errors.
E_PARSE: Errors due to incorrect syntax.
Read more on the PHP error handling manual.
Intermediate PHP Interview Questions
21. What is the difference between GET and POST methods?
GET: Delivers data in the form of a URL, limiting its length and making it visible. ideal for data retrieval.
POST: Provides more secure data by sending it in the request body. Ideal for sending private information.
Read more about GET and POST methods in PHP.
22. What is a session in PHP?
During a user's visit, data can be stored across several pages thanks to a session. It is helpful for keeping track of user preferences, shopping cart information, and login credentials. The server stores the sessions.
23. How do you start and destroy a session in PHP?
Start a session using session_start();
Destroy a session using session_destroy();
24. What is a cookie in PHP?
A cookie is a tiny bit of information that is saved on the user's browser. It aids in monitoring user preferences and behavior.
25. What is the difference between include() and require()?
include('file.php') loads a file, but if it is missing, the script continues execution.
require('file.php') loads a file, and if it is missing, the script stops with an error.
26. What is the difference between explode() and implode()?
explode() converts a string into an array based on a delimiter.
implode() joins an array into a single string using a separator.
27. What is a recursive function in PHP?
A recursive function is one that solves an issue by calling itself. It is helpful for things like factorial calculations and tree traversal.
Common use: factorial calculation in PHP.
28. What are loops in PHP?
PHP supports loops to execute a block of code multiple times:
for loop: Executes a block of code a specific number of times.
while loop: Repeats as long as a condition is true.
do-while loop: Executes at least once before checking the condition.
foreach loop: Used for iterating over arrays.
29. What is the difference between an indexed array and an associative array?
Indexed array: Uses numeric keys (e.g., [0 => 'apple', 1 => 'banana']).
Associative array: Uses named keys (e.g., ['name' => 'John', 'age' => 25]).
30. What is the use of file_get_contents() and file_put_contents()?
file_get_contents('file.txt') reads an entire file into a string.
file_put_contents('file.txt', 'Hello!') writes data to a file.
31. What is the use of mysqli_real_escape_string()?
It guards against SQL injection threats by escaping special characters in a string before utilizing them in a SQL query.
32. What are PHP sessions used for?
User data is stored across several pages using sessions. Both user preferences and authentication benefit from it.
33. What is the difference between $_SESSION and $_COOKIE?
Sessions store data on the server and are more secure.
Cookies store data on the user's browser and persist even after closing the browser.
34. What is the purpose of unset() in PHP?
A variable's value can be deleted from memory by using the unset() function.
35. What is type juggling in PHP?
PHP's ability to automatically change data types when needed—such as when converting a string to an integer for arithmetic operations—is known as type juggling.
36. What is the difference between strlen() and mb_strlen()?
strlen() counts the number of bytes in a string.
mb_strlen() correctly counts the number of characters in multi-byte encoded strings like UTF-8.
37. How do you retrieve form data using PHP?
Form data can be retrieved using $_POST (for form submissions) or $_GET (for URL parameters).
38. What is PHP magic methods?
Magic methods are special functions that begin with __ (double underscore) and are triggered automatically, such as:
__construct() – Called when an object is created.
__destruct() – Called when an object is destroyed.
39. How do you prevent cross-site scripting (XSS) attacks in PHP?
Use htmlspecialchars() or strip_tags() to sanitize user input before displaying it on a webpage.
40. What is an autoloader in PHP?
An autoloader automatically loads PHP classes when they are needed, preventing the need to manually include class files.
Advanced PHP Interview Questions
41. What is Object-Oriented Programming (OOP) in PHP?
OOP is a programming paradigm that uses classes and objects to arrange code. PHP is compatible with OOP ideas such as polymorphism, inheritance, and encapsulation.
42. What is the difference between abstract classes and interfaces?
An abstract class provides partial implementation and can have both defined and undefined methods.
An interface only contains method declarations without implementation, forcing classes to implement them.
43. What is a constructor and destructor in PHP?
A constructor (__construct()) initializes an object when it is created.
A destructor (__destruct()) cleans up resources when an object is destroyed.
44. What is PDO in PHP?
A database access layer called PDO (PHP Data Objects) supports a variety of database types and enables safe database interactions. Because of its versatility, it is favored over MySQLi.
45. How do you prevent SQL injection in PHP?
By using prepared statements and parameterized queries with PDO or MySQLi.
46. What is a PHP namespace?
Large programs can be better organized thanks to namespaces, which avoid name conflicts between classes and functions.
47. What is the clone keyword used for in PHP?
It copies an object while keeping distinct memory references.
48. How does PHP handle multiple inheritances?
Multiple inheritances are not supported by PHP; nonetheless, they are permitted through interfaces and traits.
49. What is the difference between final class and final method?
A final class cannot be extended.
A final method cannot be overridden in child classes.
50. What is the spl_autoload_register() function?
This function registers an autoloader for classes, reducing the need for multiple include statements.
Conclusion
PHP is a flexible programming language that is widely used in web development. You can show your competence and respond to interview questions with confidence if you can master these questions.
Do you want to increase your knowledge of PHP? Take the Web Development Course at IOTA Academy to gain practical experience using PHP, MySQL, and other tools to create real-world apps!📌 Begin your path to mastery of PHP right now!
Comments